Query times of valid data, hardware injections, and more.
Information about the state of the LIGO/Virgo data can be queried from
resources at the GWOSC and from LVC-proprietary DQSEGDB through the
functions of the pycbc.dq
module. We will outline a few of common
tasks below.
Determine the times an instrument has valid data
"""This example shows how to determine when a detector is active."""
import matplotlib.pyplot as pp
from pycbc import dq
from pycbc.results import ifo_color
start_time = 1126051217
end_time = start_time + 100000
# Get times that the Hanford detector has data
hsegs = dq.query_flag('H1', 'DATA', start_time, end_time)
# Get times that the Livingston detector has data
lsegs = dq.query_flag('L1', 'DATA', start_time, end_time)
pp.figure(figsize=[10,2])
for seg in lsegs:
start, end = seg
pp.axvspan(start, end, color=ifo_color('L1'), ymin=0.1, ymax=0.4)
for seg in hsegs:
start, end = seg
pp.axvspan(start, end, color=ifo_color('H1'), ymin=0.6, ymax=0.9)
pp.xlabel('Time (s)')
pp.show()
(Source code
, png
, hires.png
, pdf
)
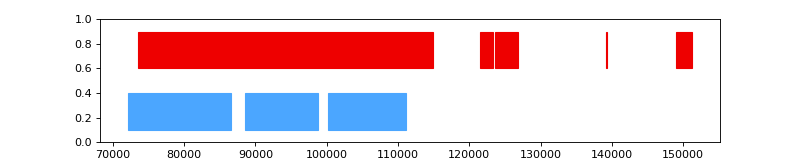
Finding times of hardware injections
"""This example shows how to determine when a CBC hardware injection is present
in the data from a detector.
"""
import matplotlib.pyplot as pp
from pycbc import dq
start_time = 1126051217
end_time = start_time + 10000000
# Get times that the Livingston detector has CBC injections into the data
segs = dq.query_flag('L1', 'CBC_HW_INJ', start_time, end_time)
pp.figure(figsize=[10, 2])
for seg in segs:
start, end = seg
pp.axvspan(start, end, color='blue')
pp.xlabel('Time (s)')
pp.show()
(Source code
, png
, hires.png
, pdf
)
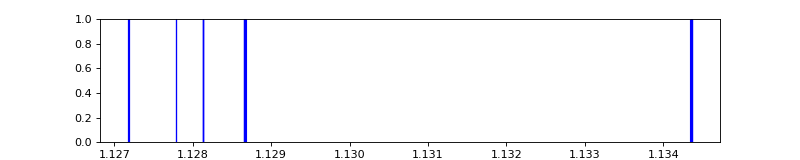
What flags can I query?
A list of many of the flags which can be quiered is available here. Instead, just give the raw name such as “DATA” instead of “H1_DATA”.
There are two additional types of flags which can be queried. These are the negation of the flags like “NO_CBC_HW_INJ”. The flag “CBC_HW_INJ” would give times where there is a hardware injection instead of times when there isn’t. Similarly if you use “CBC_CAT2_VETO” instead of “CBC_CAT2” you will get the times that are adversely affected instead of the times that are not.